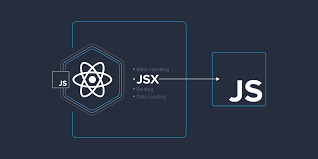
What is JSX?
JSX (JavaScript XML) is a syntax extension for JavaScript used in React to describe the UI structure in a syntax similar to HTML. It allows developers to write HTML-like code directly within JavaScript files, making it easier to create and visualize the UI components.
JSX is not a separate language; it is syntactic sugar over the React.createElement() function. When a JSX expression is compiled, it gets converted into JavaScript code that React understands.
For example:
const element = <h1>Hello, World!</h1>;
is transformed into:
const element = React.createElement("h1", null, "Hello, World!");
Why Use JSX in React?
JSX provides several advantages when working with React:
- Improved Readability and Maintainability
- JSX makes the code more readable by resembling HTML, which is familiar to web developers.
- It allows developers to structure UI components in a way that visually represents the final output.
- Boosts Developer Productivity
- Writing UI components in JSX is faster compared to using
React.createElement()
manually. - JSX allows embedding JavaScript expressions using curly braces (
{}
), making it dynamic and flexible.
- Better Error Detection
- JSX provides compile-time error checking, reducing runtime errors and making debugging easier.
- Integration with React’s Virtual DOM
- JSX works seamlessly with React’s Virtual DOM, ensuring efficient UI updates and rendering.
- Encapsulation of UI Components
- JSX allows combining HTML and JavaScript logic inside React components, promoting modularity and reusability.
JSX vs Regular JavaScript
Example Comparison
Using JSX
const Greeting = () => { return <h1>Hello, React!</h1>; };
Using Regular JavaScript
const Greeting = () => { return React.createElement("h1", null, "Hello, React!"); };
Conclusion
JSX is a powerful and intuitive way to write UI components in React. While it needs to be compiled to JavaScript before execution, it significantly enhances developer productivity, readability, and maintainability. Understanding JSX is crucial for working efficiently with React and building scalable applications.
Leave a Comment